Working on a large iOS codebase often involves a lot of waiting: Waiting for Xcode to index your files, waiting for Swift and Objective-C code to compile, waiting for the Simulator to boot and your app to launch…
- Mac Catalina Xcode Version
- Mac Catalina Xcode Command Line Tools
- Mac Catalina Xcode 10
- Mac Os Catalina Xcode Command Line Tools
Many Catalina directories are not writable by the old tool installer. XProtect prevents modification. The newest Xcode installer puts stuff in other places (not /System). Old tools may require using old OS volumes. – hotpaw2 Feb 18 '20 at 16:49. Install XCode on macOS Catalina 10.15 on Windows PC. When you installed macOS Catalina 10.15 on Virtual machine. Then Power on the virtual machine. When you entered the macOS Catalina window click on the Apple Mac App Store. Once the App Store opened on the left-handed search bar type XCode and hit enter. Xcode Update Mac Catalina The Assistant button splits the Xcode editor in two, with your primary work document on the left and an intelligent Assistant editor pane to the right. The Assistant editor automatically displays files that Xcode determines are most helpful to you based on the work you are performing in the primary editor. Just as with macOS 10.14, the 10.15 SDK does not support building 32-bit code, and furthermore 32-bit programs cannot run on Catalina. Any ports of software that lacks 64-bit support or needs to be universal will thus fail to build or otherwise encounter problems on Catalina. Most of these will not be able to be fixed short of adding 64-bit.
And after all of that, you spend even more time getting your app into a particular state and onto a particular screen, just to see whether the Auto Layout constraint you just added fixes that regression you found. It didn’t, of course, so you jump back into Xcode, tweak the Content Hugging Priority, hit ⌘R, and start the whole process again.
We might relate our sorry predicament to that one xkcd comic, but for those of us who don’t so much relish in the stop-and-go nature of app development, there’s an old Yiddish joke about Shlemiel the painter (provided below with a few -specific modifications; for the uninitiated, please refer to Joel Spolsky’s original telling):
Shlemiel gets a job as a software developer, implementing a new iOS app. On the first sprint he opens Xcode and implements 10 new screens of the app. “That’s pretty good!” says his manager, “you’re a fast worker!” and pays him a Bitcoin.
The next sprint Shlemiel only gets 5 screens done. “Well, that’s not nearly as good as yesterday, but you’re still a fast worker. 5 screens is respectable,” and pays him a Bitcoin.
The next sprint Shlemiel implements 1 screen. “Only 1!” shouts his manager. “That’s unacceptable! On the first day you did ten times that much work! What’s going on?”
“I can’t help it,” says Shlemiel. “Each sprint I get further and further away from application(_:didFinishLaunchingWithOptions:)
!”
Over the years, there have been some developments that’ve helped things slightly, including @IBInspectable
and @IBDesignable
and Xcode Playgrounds. But with Xcode 11, our wait is finally over — and it’s all thanks to SwiftUI.
The functionality described in this article requires the following:
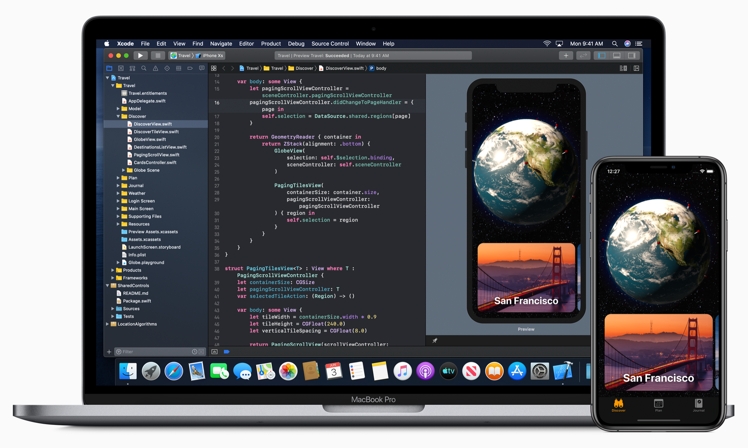
- Xcode 11
- macOS Catalina
- iOS 13 set as the Deployment Target for your app’s Debug configuration
(In Xcode, navigate your project’s Build Settings; under the Deployment heading, expand the iOS Deployment Target setting and set Debug to iOS 13.0 or later)
Without these three things, your code either won’t compile or won’t render live previews.
Although many of us have taken a “wait and see” approach to SwiftUI, we can start using its capabilities today to radically speed up and improve our development process — without changing a line of code in our UIKit apps.
Consider a subclass of UIButton
that draws a border around itself:
Normally, if we wanted to test how our UI element performs, we’d have to add it to a view in our app, build and run, and navigate to that screen. But with Xcode 11, we can now see a preview side-by-side with the code editor by adding the following under the original declaration of BorderedButton
:
Using a new feature called dynamic replacement, Xcode can update this preview without recompiling — within moments of your making a code change. This lets you rapidly prototype changes like never before.
Want to see how your button handles long titles? Bang away on your keyboard within the call to setTitle(_:for:)
in your preview, and test out potential fixes in your underlying implementation without so much as leaving your current file!
UIViewPreview
is a custom, generic structure that we created to conveniently host previews of UIView
subclasses. Feel free to download the source and add it to your project directly.
Incorporating a proper dependency would be complicated by the conditional import and iOS 13 Deployment Target settings required to make Xcode Previews work for non-SwiftUI apps, so in this particular instance, we think it’s best to embed these files directly.
Expand for the full implementation of UIViewPreview
:
Previewing Multiple States
Let’s say our app had a FavoriteButton
—a distant cousin (perhaps by composition) to BorderedButton
.In its default state,it shows has the title “Favorite”and displays a ♡ icon.When its isFavorited
property is set to true
,the title is set to “Unfavorite”and displays a ♡̸ icon.
We can preview both at once by wrapping two UIViewPreview
instances within a single SwiftUI Group
:
The chained previewLayout
and padding
methods apply to each member of the Group
. You can use these and other View
methods to change the appearance of your previews.
Previewing Dark Mode
With Dark Mode in iOS 13, it’s always a good idea to double-check that your custom views are configured with dynamic colors or accommodate both light and dark appearance in some other way.
An easy way to do this would be to use a ForEach
elementto render a preview for each case in the ColorScheme
enumeration:
When rendering previews with ForEach
, use the previewDisplayName
method to help distinguish amongall of the enumerated values.
Previewing Dynamic Type Size Categories
We can use the same approach to preview our views in various Dynamic Type Sizes:
Previewing Different Locales
Xcode Previews are especially time-saving when it comes to localizing an app into multiple languages. Compared to the hassle of configuring Simulator back and forth between different languages and regions, this new approach makes a world of difference.
Let’s say that, in addition to English, your app supported various right-to-left languages. You could verify that your RTL logic worked as expected like so:
We don’t know of an easy way to use NSLocalizedString
with an explicit locale. You could go to the trouble of retrieving localized strings from a strings file in your bundle, but in most cases, you’ll be just fine hard-coding text in your previews.
Previewing View Controllers on Different Devices
SwiftUI previews aren’t limited to views, you can also use them with view controllers. By creating a custom UIViewControllerPreview
typeand taking advantage of somenew UIStoryboard
class methods in iOS 13,we can easily preview our view controlleron various devices —one on top of another:
There’s currently no way to get SwiftUI device previews in landscape orientation. Although you can approximate this with a fixed size preview layout, be aware that it won’t respect Safe Area on iPhone or render split views correctly on iPad.
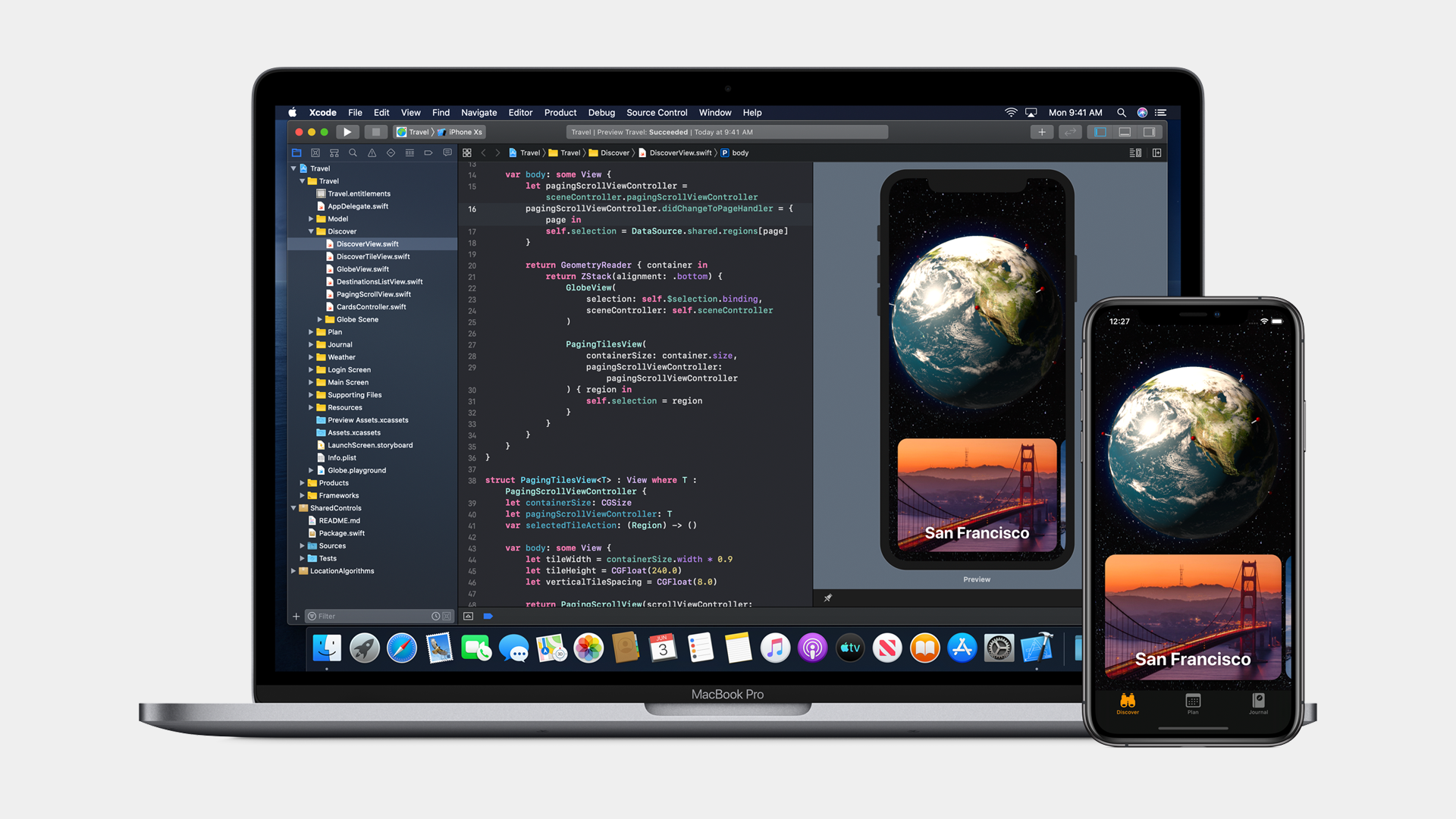
Although most of us are still some years away from shipping SwiftUI in our apps (whether by choice or necessity), we can all immediately benefit from the order-of-magnitude improvement it enables with Xcode 11 on macOS Catalina.
By eliminating so much time spent waiting for things to happen, we not only get (literally) hours more time each week, but we unlock the possibility of maintaining an unbroken flow state during that time. Not only that, but the convenience of integrated tests fundamentally changes the calculus for testing: instead of being a rare “nice to have,” they’re the new default. Plus: these inline previews serve as living documentation that can help teams both large and small finally get a handle on their design system.
It’s hard to overstate how much of a game-changer Xcode Previews are for iOS development, and we couldn’t be happier to incorporate them into our workflow.
Xcode is the tool developers use to build apps for the Apple ecosystem – MacOS, iOS, and all things Apple.
This guide will walk you through how to successfully install Xcode onto your Mac, from start to finish.
Here are some handy tips to know before you get started:
- Xcode only runs on a mac. If you are on a PC, sadly you won't be able to use Xcode.
- You'll need a good, stable internet connection. The latest version is around 8 gigabytes in size.
- Be sure to have at least 30 gigabytes of free space on your computer. The latest
.xip
file (v11.4.1 at the time of writing) is ~8 gigabytes zipped. When you unzip it, that's another 17 gigabytes. Then you'll need the command line tool, which is yet another 1.5 gigabytes.
Here's an overview of the steps to install Xcode


- Download Xcode
- Install the command line tool
- Open the new version
- Delete files
Note that I have listed some Terminal commands in the steps below. These commands can be typed into your present working directory. This means that you don't need to navigate to any particular folder.
If you really want to, you can first type cd
before typing the commands in the below steps. This will return you back to the home folder.
Step #1: Download Xcode
There are two ways to do this. For the latest version and a theoretically 'easy' installation, you can use the App Store. I don't recommend this option.
I prefer to use the developer site. This comes with the bonus option of being able to download any version you'd like.
Option #1: Download via the App Store for the latest version (not my preferred option)
In theory, this should be a seamless and pain-free process. But if the installation fails for any reason on the last step, it is very hard to troubleshoot.
There are a few reasons for failure, and no easy way to know which is the underlying cause. If you do encounter a failure, you will need to re-download the entire file again each time you try to fix the failure. As the latest version is 8 gigabytes, I didn't much enjoy this approach.
But if you're feeling brave, here are the steps:
- Open the App Store on your mac
- Sign in
- Search for Xcode
- Click install or update
Option 2: Download via the Developer site for a specific version (my preferred option)
- Head to the 'more' section of the Apple developer website
- Sign in with your iTunes account id
- Type in the version that you'd like, and download the
Xcode_x_x_x.xip
file. Keep in mind that Xcode 11.4.1 is 8 gigabytes, so this will take awhile depending on your internet connection. - Once the file is downloaded, click on
.xip
to extract it. Your laptop will extract it to the same folder you downloaded it to. This extraction process is automatic. You don't need to do anything more after you click on the.xip
file. This step will take a few minutes. - [Optional] Once extracted, rename the application to “Xcode11.x.x” if you are using multiple versions.
- Drag application to the Applications folder
- [Optional] Set the new Xcode version as the default. Open Terminal and type
sudo xcode-select -switch /Applications/Xcodex.x.x.app
. Replacex.x.x
with the version number. For example:Xcode11.4.1.app
. You will need to enter in your computer admin password. I'm pretty sure this will update the default Xcode version for all users on your computer, so best to check with other users first
Step #2: Install the command line tool (CLT)
If you have multiple users on your computer, you will need to update the CLT for each user.
Download .dmg
To update the CLT, go to app developer website and download the command line tool .dmg
.
If you have never installed Xcode before, you may be able to update with your Terminal by typing in xcode-select --install
instead of visiting the developer website.
But if you have an existing version of Xcode installed on your machine, you'll probably see this error:
This means you'll need to go to the developer website instead.
Installing the CLT
When the .dmg
has finished downloaded, double click the file to open it. This will open a little window that looks like this:
Double click the box and follow the prompts to install the CLT. It will take a few minutes to complete.
It may ask you at the end of the installation whether you want to move this to the trash bin. When it does this, it's talking about moving the .dmg
file to the trash bin. Since you should no longer need this file. I always say yes to this.
Step #3: Open Xcode
Mac Catalina Xcode Version
Open the Applications folder and open the new version of Xcode. If you renamed Xcode, make sure you open the correct application
Xcode may prompt you to install additional components. Click install. This will take a few minutes.
While it's installing, check that your default Xcode version is the one you just downloaded:
- Open Terminal
- Type
brew config
- You should see “CLT” and “Xcode” versions, as well as everything else. This should reflect the version that you have just downloaded. In my case, I downloaded Xcode 11.4.1.
Once the components are installed, Xcode will launch. You should be able to pick up your old projects and continue where you left off seamlessly*.
*Note that if you use any proxy tools, such as Charles, you will need to re-install those certificates in your simulator again.
If you encounter any errors while trying to build or run a project, check which device you are trying to launch. Bird photo booth pictures on. The new version may not remember the device you were using before. If so, click on the device and choose 'Add additional simulators' from the drop down menu to add the device you want.
Mac Catalina Xcode Command Line Tools
Step #4. Delete the files
If you don't need the older versions of Xcode on your computer, you can uninstall them and get some hard drive space back.
Mac Catalina Xcode 10
You can also delete the .xip
file of the version you just downloaded, as well as the CLT.dmg
file.
Mac Os Catalina Xcode Command Line Tools
That's everything. I hope this has helped you successfully install Xcode. Have fun with it!